|
1 | 1 | # RGB.NET
|
| 2 | +[](https://github.com/DarthAffe/RGB.NET/releases) |
| 3 | +[](https://www.nuget.org/packages?q=rgb.net) |
| 4 | +[](https://github.com/DarthAffe/RGB.NET/blob/master/LICENSE) |
| 5 | +[](https://github.com/DarthAffe/RGB.NET/stargazers) |
| 6 | +[](https://discord.gg/9kytURv) |
2 | 7 |
|
3 |
| -This project aims to unify the use of various RGB-devices. |
4 |
| -**It is currently under heavy development and will have breaking changes in the future!** Right now a lot of devices aren't working as expected and there are bugs/unfinished features. Please think about that when you consider using the library in this early stage. |
5 |
| - |
6 |
| -If you want to help with layouting/testing devices or if you need support using the library feel free to join the [RGB.NET discord-channel](https://discord.gg/9kytURv). |
| 8 | +> **IMPORTANT NOTE** |
| 9 | +This is a library to integrate RGB-devices into your own application. It does not contain any executables! |
| 10 | +If you're looking for a full blown software solution to manage your RGB-devices, take a look at [Artemis](https://artemis-rgb.com/). |
7 | 11 |
|
| 12 | +## Getting Started |
| 13 | +### Setup |
| 14 | +1. Add the [RGB.NET.Core](https://www.nuget.org/packages/RGB.NET.Core) and [Devices](https://www.nuget.org/packages?q=rgb.net.Devices)-Nugets for all devices you want to use. |
| 15 | +2. For some of the vendors SDK-libraries are needed. Check the contained Readmes for more information in that case. |
| 16 | +3. Create a new `RGBSurface`. |
| 17 | +```csharp |
| 18 | +RGBSurface surface = new RGBSurface(); |
| 19 | +``` |
8 | 20 |
|
9 |
| -## Adding prerelease packages using NuGet ## |
10 |
| -This is the easiest and therefore preferred way to include RGB.NET in your project. |
| 21 | +4. Initialize the providers for all devices you want to use and add the devices to the surface. For example: |
| 22 | +```csharp |
| 23 | +CorsairDeviceProvider.Instance.Initialize(throwExceptions: true); |
| 24 | +surface.Attach(CorsairDeviceProvider.Instance.Devices); |
| 25 | +``` |
| 26 | +The `Initialize`-method allows to load only devices of specific types by setting a filter and for debugging purposes allows to enable exception throwing. (By default they are catched and provided through the `Exception`-event.) |
| 27 | +You can also use the `Load`-Extension on the surface. |
| 28 | +```csharp |
| 29 | +surface.Load(CorsairDeviceProvider.Instance); |
| 30 | +``` |
| 31 | +> While most device-providers are implemented in a way that supports fast loading like this some may have a different loading procedures. (For example the `WS281XDeviceProvider` requires device-definitions before loading.) |
11 | 32 |
|
12 |
| -Since there aren't any release-packages right now you'll have to use the CI-feed from [http://nuget.arge.be](http://nuget.arge.be). |
13 |
| -You can include it either by adding ```http://nuget.arge.be/v3/index.json``` to your Visual Studio package sources or by adding this [NuGet.Config](https://github.com/DarthAffe/RGB.NET/tree/master/Documentation/NuGet.Config) to your project (at the same level as your solution). |
| 33 | +5. Add an update-trigger. In most cases the TimerUpdateTrigger is preferable, but you can also implement your own to fit your needs. |
| 34 | +```csharp |
| 35 | +surface.RegisterUpdateTrigger(new TimerUpdateTrigger()); |
| 36 | +``` |
| 37 | +> If you want to trigger updates manually the `ManualUpdateTrigger` should be used. |
14 | 38 |
|
15 |
| -### .NET 4.5 Support ### |
16 |
| -At the end of the year with the release of .NET 5 the support for old .NET-Framwork versions will be droppped! |
17 |
| -It's not recommended to use RGB.NET in projects targeting .NET 4.x that aren't planned to be moved to Core/.NET 5 in the future. |
| 39 | +6. *This step is optional but recommended.* For rendering the location of each LED on the surface can be important. Since not all SDKs provide useful layout-information you might want to add Layouts to your devices. (TODO: add wiki article for this) |
| 40 | +Same goes for the location of the device on the surface. If you don't care about the exact location of the devices you can use: |
| 41 | +```csharp |
| 42 | +surface.AlignDevices(); |
| 43 | +``` |
18 | 44 |
|
| 45 | +The basic setup is now complete and you can start setting up your rendering. |
19 | 46 |
|
20 |
| -### Device-Layouts |
21 |
| -To be able to have devices with correct LED-locations and sizes they need to be layouted. Pre-created layouts can be found at https://github.com/DarthAffe/RGB.NET-Resources. |
| 47 | +### Basic Rendering |
| 48 | +As an example we'll add a moving rainbow over all devices on the surface. |
| 49 | +1. Create a led-group containing all leds on the surface (all devices) |
| 50 | +```csharp |
| 51 | +ILedGroup allLeds = new ListLedGroup(surface, surface.Leds); |
| 52 | +``` |
22 | 53 |
|
23 |
| -If you plan to create layouts for your own devices check out https://github.com/DarthAffe/RGB.NET/wiki/Creating-Layouts first. There's also a layout-editor which strongly simplifies most of the work: https://github.com/SpoinkyNL/RGB.NET-Layout-Editor |
| 54 | +2. Create a rainbow-gradient. |
| 55 | +```csharp |
| 56 | +RainbowGradient rainbow = new RainbowGradient(); |
| 57 | +``` |
24 | 58 |
|
25 |
| -### Example usage of RGB.NET |
26 |
| -[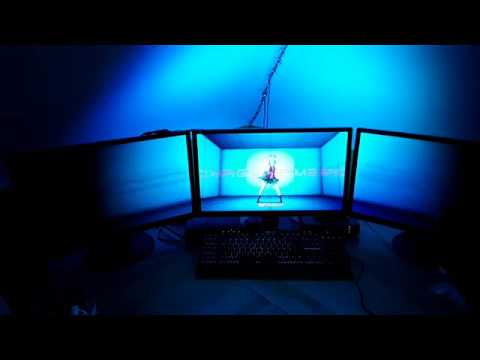](http://www.youtube.com/watch?v=JLRa0Wv4qso) |
| 59 | +3. Add a decorator to the gradient to make it move. (Decorators are |
| 60 | +```csharp |
| 61 | +rainbow.AddDecorator(new MoveGradientDecorator(surface)); |
| 62 | +``` |
27 | 63 |
|
28 |
| -#### Example Projects |
29 |
| -[https://github.com/DarthAffe/KeyboardAudioVisualizer](https://github.com/DarthAffe/KeyboardAudioVisualizer) |
30 |
| -[https://github.com/DarthAffe/RGBSyncPlus](https://github.com/DarthAffe/RGBSyncPlus) |
| 64 | +4. Create a texture (the size - in this example 10, 10 - is not important here since the gradient shoukd be stretched anyway) |
| 65 | +```csharp |
| 66 | +ITexture texture = new ConicalGradientTexture(new Size(10, 10), rainbow); |
| 67 | +``` |
| 68 | + |
| 69 | +5. Add a brush rendering the texture to the led-group |
| 70 | +```csharp |
| 71 | +allLeds.Brush = new TextureBrush(texture); |
| 72 | +``` |
| 73 | + |
| 74 | +### Full example |
| 75 | +```csharp |
| 76 | +RGBSurface surface = new RGBSurface(); |
| 77 | +surface.Load(CorsairDeviceProvider.Instance); |
| 78 | +surface.AlignDevices(); |
| 79 | + |
| 80 | +surface.RegisterUpdateTrigger(new TimerUpdateTrigger()); |
| 81 | + |
| 82 | +ILedGroup allLeds = new ListLedGroup(surface, surface.Leds); |
| 83 | +RainbowGradient rainbow = new RainbowGradient(); |
| 84 | +rainbow.AddDecorator(new MoveGradientDecorator(surface)); |
| 85 | +ITexture texture = new ConicalGradientTexture(new Size(10, 10), rainbow); |
| 86 | +allLeds.Brush = new TextureBrush(texture); |
| 87 | +``` |
0 commit comments