|
6 | 6 |
|
7 | 7 | ## What is OSLC4NET?
|
8 | 8 |
|
9 |
| -**OSLC4Net** is an SDK and sample applications that help the .NET community adopt Open Services for Lifecycle Collaboration (OSLC, [homepage](http://open-services.net)) and build OSLC-conformant tools. |
10 |
| - |
11 |
| -The SDK allows developers to create OSLC servers and clients by adding OSLC annotations to .NET objects to represent them as OSLC resources. It includes a library based on the [dotNetRDF](https://dotnetrdf.org/) package, which assists with representing these resources as RDF and helps parse Turle, RDF/XML, and JSON-LD documents into OSLC .NET objects. |
12 |
| - |
13 |
| -The [OSLC4Net.Client package](https://www.nuget.org/packages/OSLC4Net.Client/) can be used to help create consumer REST requests. On the server side, the project offers an RDF-specific `MediaTypeFormatter` that can help process OSLC REST requests within an ASP.NET MVC 5 API. **Join the [discussion on the .NET Core migration](https://github.com/OSLC/oslc4net/issues/25).** |
| 9 | +**OSLC4Net** is an SDK and sample applications that help the .NET community |
| 10 | +adopt Open Services for Lifecycle Collaboration (OSLC, |
| 11 | +[homepage](http://open-services.net)) and build OSLC-conformant tools. |
| 12 | + |
| 13 | +The SDK allows developers to create OSLC servers and clients by adding OSLC |
| 14 | +annotations to .NET objects to represent them as OSLC resources. It includes a |
| 15 | +library based on the [dotNetRDF](https://dotnetrdf.org/) package, which assists |
| 16 | +with representing these resources as RDF and helps parse Turle, RDF/XML, and |
| 17 | +JSON-LD documents into OSLC .NET objects. |
| 18 | + |
| 19 | +The [OSLC4Net.Client package](https://www.nuget.org/packages/OSLC4Net.Client/) |
| 20 | +can be used to help create consumer REST requests. On the server side, the |
| 21 | +project offers an RDF-specific `MediaTypeFormatter` that can help process OSLC |
| 22 | +REST requests within an ASP.NET MVC 5 API. **Join the [discussion on the .NET |
| 23 | +Core migration](https://github.com/OSLC/oslc4net/issues/25).** |
14 | 24 |
|
15 | 25 | ## Getting started
|
16 | 26 |
|
17 |
| -If you do not have a .NET development environment, start by downloading VS Code [C# Dev Kit](https://marketplace.visualstudio.com/items?itemName=ms-dotnettools.csdevkit). Make sure to install .NET 8 SDK for development. Libraries target NETStandard 2.0 and should run on .NET Framework 4.8 or .NET 6+ (recommended). |
| 27 | +If you do not have a .NET development environment, start by downloading VS Code |
| 28 | +[C# Dev |
| 29 | +Kit](https://marketplace.visualstudio.com/items?itemName=ms-dotnettools.csdevkit). |
| 30 | +Make sure to install .NET 8 SDK for development. Libraries target NETStandard |
| 31 | +2.0 and should run on .NET Framework 4.8 or .NET 6+ (recommended). |
18 | 32 |
|
19 | 33 | ### A simple OSLC Client
|
20 | 34 |
|
21 |
| -Create a new console application targeting .NET 6+, add a NuGet dependency to `OSLC4Net.Client` (make sure the *Include prerelease* is checked if you see an empty list) and add the following code: |
| 35 | +Create a new console application targeting .NET 6+, add a NuGet dependency to |
| 36 | +`OSLC4Net.Client` (make sure the *Include prerelease* is checked if you see an |
| 37 | +empty list) and add the following code: |
22 | 38 |
|
23 | 39 | ```csharp
|
24 |
| -private const string OSLC_SERVER_URI = "https://oslc.itm.kth.se/ccm"; |
| 40 | +var oslcClient = OslcClient.ForBasicAuth(username, password); |
25 | 41 |
|
26 |
| -static void Main(string[] args) |
| 42 | +var resourceUri = |
| 43 | + "https://jazz.net/sandbox01-ccm/resource/itemName/com.ibm.team.workitem.WorkItem/1300"; |
| 44 | +OslcResponse<ChangeRequest> response = await oslcClient.GetResourceAsync<ChangeRequest>(resourceUri); |
| 45 | +if (response.Resource is not null) |
27 | 46 | {
|
28 |
| - try |
29 |
| - { |
30 |
| - var helper = new JazzRootServicesHelper(OSLC_SERVER_URI, OSLCConstants.OSLC_CM_V2); |
31 |
| - var catUri = helper.GetCatalogUrl(); |
32 |
| - Console.WriteLine($"The OSLC server has an OSLC Service Provider Catalog at the following URI:\n {catUri}"); |
33 |
| - } catch (RootServicesException e) { |
34 |
| - Console.WriteLine($"Failed to fetch the OSLC RootServices document from:\n {OSLC_SERVER_URI}/rootservices"); |
35 |
| - } |
36 |
| - Console.ReadLine(); |
| 47 | + ChangeRequest wi1300 = response.Resource; |
| 48 | + logger.LogInformation($"{wi1300.GetShortTitle()} {wi1300.GetTitle()}"); |
| 49 | +} |
| 50 | +else |
| 51 | +{ |
| 52 | + logger.LogError("Something went wrong: {} {}", (response.StatusCode as int?) ?? -1, |
| 53 | + response.ResponseMessage?.ReasonPhrase); |
37 | 54 | }
|
38 |
| -``` |
39 |
| - |
40 |
| -This should give you a valid response. |
41 |
| - |
42 |
| -### Running the sample StockQuote provider |
43 |
| - |
44 |
| -> [!NOTE] |
45 |
| -> Sample StockQuote provider has not yet been migrated to .NET 6+. |
46 |
| -
|
47 |
| -> [!WARNING] |
48 |
| -> Sample StockQuote provider is a toy implementation and does not correspond to any [standards-track OSLC specifications](https://open-services.net/specifications/). |
49 |
| -
|
50 |
| -`OSLC4Net.StockQuoteSample` is a sample OSLC provider which implements one resource type, a StockQuote. This resource is not defined by an OSLC specification, it shows how OSLC4Net can be used to create an experimental OSLC provider. |
51 | 55 |
|
52 |
| -1. Build the `OSLC4Net_SDK\OSLC4Net.Core.sln` solution |
53 |
| -1. Right click the `OSLC4Net.StockQuoteSample` project and run it via _Debug->Start new instance_ |
| 56 | +``` |
54 | 57 |
|
55 |
| -You'll see a web page created - that is currently just a skeleton provided by ASP.NET. Try performing a GET request using [Postman](https://www.postman.com/) (make sure to set the `Accept` header to `application/rdf+xml`: |
| 58 | +Replace `resourceUri` with a valid OSLC resource URI. This should give you a |
| 59 | +valid response. See [full example |
| 60 | +project](./OSLC4Net_SDK/Examples/Oslc4NetExamples.Client/) for more details. |
56 | 61 |
|
57 |
| -* http://localhost:7077/api/stockquote - returns all StockQuotes |
58 |
| -* http://localhost:7077/api/stockquote?getShape=true - returns the StockQuote OSLC resource shape |
59 |
| -* http://localhost:7077/api/stockquote/nasdaq_goog - returns an individual StockQuote |
| 62 | +> [!TIP] |
| 63 | +> |
| 64 | +> Use https://github.com/oslc-op/refimpl to quickly run a few conformant OSLC |
| 65 | +> servers. |
60 | 66 |
|
61 |
| -A request to the first URL using Fiddler should have the following response: |
| 67 | +## OSLC Server support |
62 | 68 |
|
63 |
| -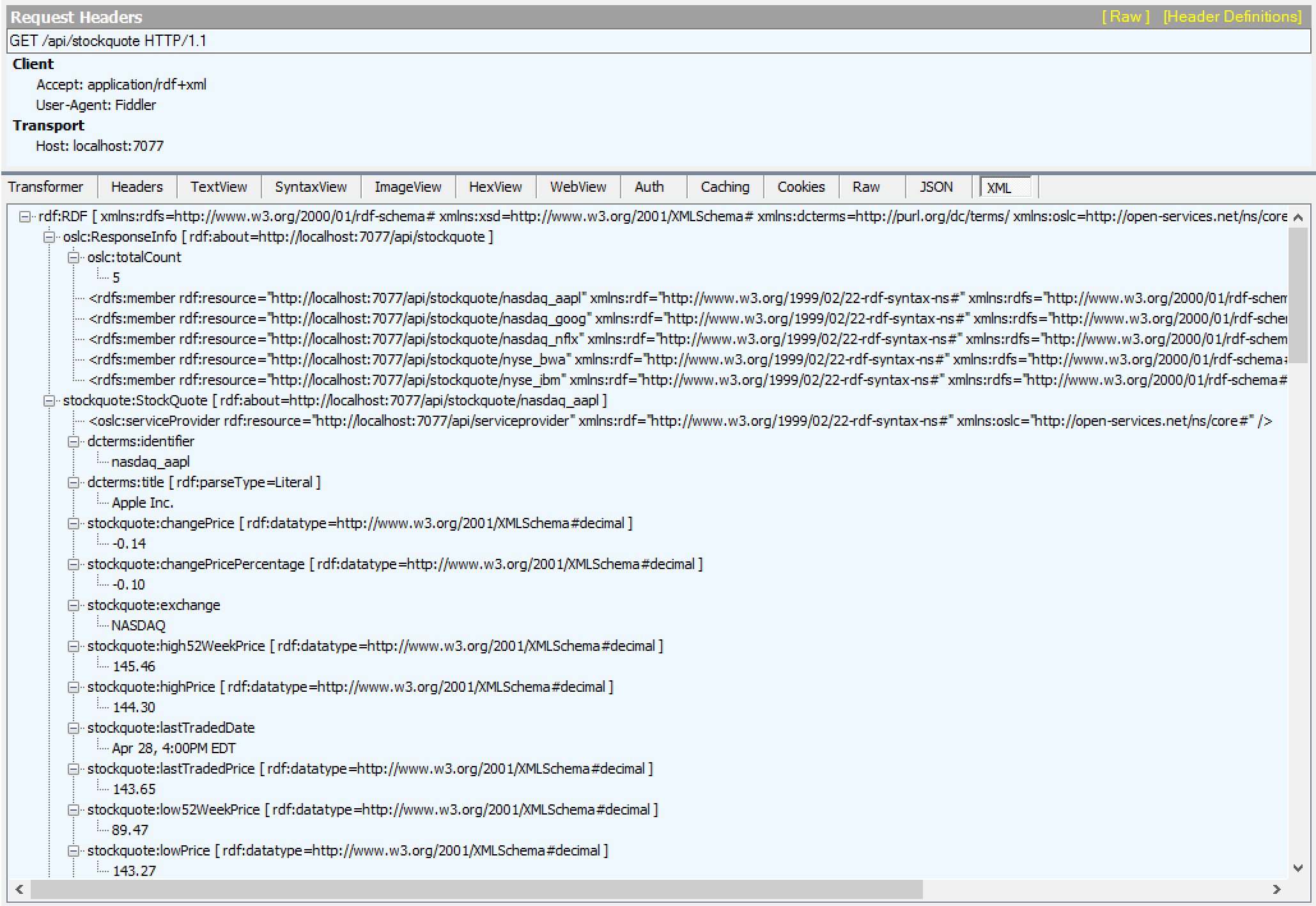 |
| 69 | +Server parts of the SDK have not yet been migrated from .NET Framework to .NET |
| 70 | +8+. |
64 | 71 |
|
65 | 72 | ## More information on OSLC
|
66 | 73 |
|
67 |
| -* See the [OSLC](http://open-services.net/) site for more details on OSLC specifications and community activities. |
68 |
| -* See the [Eclipse Lyo](http://eclipse.org/lyo) site for information on OSLC SDKs and samples for other technologies. |
| 74 | +* See the [OSLC](http://open-services.net/) site for more details on OSLC |
| 75 | + specifications and community activities. |
| 76 | +* See the [Eclipse Lyo](http://eclipse.org/lyo) site for information on OSLC |
| 77 | + SDKs and samples for other technologies. |
69 | 78 |
|
70 | 79 | ## OSLC4Net License
|
71 | 80 |
|
|
0 commit comments