|
| 1 | +--- |
| 2 | +title: How to set up a React Native remote config (with Expo Router) |
| 3 | +date: 2025-02-06 |
| 4 | +author: |
| 5 | + - ian-vanagas |
| 6 | +tags: |
| 7 | + - feature flags |
| 8 | +--- |
| 9 | + |
| 10 | +[Remote config](/docs/feature-flags/remote-config) enables you to update your React Native app's settings and behavior instantly without deploying new code or waiting for app store approval. This makes it perfect for controlling features on the fly and disabling problematic features if needed. |
| 11 | + |
| 12 | +This tutorial shows you how to set up remote config in your React Native app using PostHog and Expo Router. We'll create a basic app that displays a message which we'll control using remote config. |
| 13 | + |
| 14 | +## 1. Creating a new React Native app |
| 15 | + |
| 16 | +First, let's create a new React Native app using the Expo Router template. This assumes you have [Node](https://nodejs.org/en/) installed and the Expo Go app on either [Android](https://play.google.com/store/apps/details?id=host.exp.exponent) or [iOS](https://apps.apple.com/app/expo-go/id982107779). |
| 17 | + |
| 18 | +```bash |
| 19 | +npx create-expo-app@latest rn-remote-config |
| 20 | +cd rn-remote-config |
| 21 | +``` |
| 22 | + |
| 23 | +After this, run `npx expo start` and open it with Expo Go to see your app in action. |
| 24 | + |
| 25 | +## 2. Adding PostHog to your app |
| 26 | + |
| 27 | +With our app structure set up, let's install and configure PostHog. If you don't have a PostHog instance, you can [sign up for free](https://us.posthog.com/signup). |
| 28 | + |
| 29 | +To start, install PostHog's React Native SDK and its dependencies: |
| 30 | + |
| 31 | +```bash |
| 32 | +npx expo install posthog-react-native expo-file-system expo-application expo-device expo-localization |
| 33 | +``` |
| 34 | + |
| 35 | +Next, update your root layout file (`app/_layout.tsx`) to include the `PostHogProvider` set up with your project's API key and client host which you can find in [your project settings](https://us.posthog.com/settings/project). |
| 36 | + |
| 37 | +```tsx |
| 38 | +import { DarkTheme, DefaultTheme, ThemeProvider } from '@react-navigation/native'; |
| 39 | +import { useFonts } from 'expo-font'; |
| 40 | +import { Stack } from 'expo-router'; |
| 41 | +import * as SplashScreen from 'expo-splash-screen'; |
| 42 | +import { StatusBar } from 'expo-status-bar'; |
| 43 | +import { useEffect } from 'react'; |
| 44 | +import 'react-native-reanimated'; |
| 45 | +import { PostHogProvider } from 'posthog-react-native' |
| 46 | + |
| 47 | +import { useColorScheme } from '@/hooks/useColorScheme'; |
| 48 | + |
| 49 | +// Prevent the splash screen from auto-hiding before asset loading is complete. |
| 50 | +SplashScreen.preventAutoHideAsync(); |
| 51 | + |
| 52 | +export default function RootLayout() { |
| 53 | + const colorScheme = useColorScheme(); |
| 54 | + const [loaded] = useFonts({ |
| 55 | + SpaceMono: require('../assets/fonts/SpaceMono-Regular.ttf'), |
| 56 | + }); |
| 57 | + |
| 58 | + useEffect(() => { |
| 59 | + if (loaded) { |
| 60 | + SplashScreen.hideAsync(); |
| 61 | + } |
| 62 | + }, [loaded]); |
| 63 | + |
| 64 | + if (!loaded) { |
| 65 | + return null; |
| 66 | + } |
| 67 | + |
| 68 | + return ( |
| 69 | + <PostHogProvider |
| 70 | + apiKey="<ph_project_api_key>" |
| 71 | + options={{ |
| 72 | + host: "<ph_client_api_host>", // usually 'https://us.i.posthog.com' or 'https://eu.i.posthog.com' |
| 73 | + }} |
| 74 | + > |
| 75 | + <ThemeProvider value={colorScheme === 'dark' ? DarkTheme : DefaultTheme}> |
| 76 | + <Stack> |
| 77 | + <Stack.Screen name="(tabs)" options={{ headerShown: false }} /> |
| 78 | + <Stack.Screen name="+not-found" /> |
| 79 | + </Stack> |
| 80 | + <StatusBar style="auto" /> |
| 81 | + </ThemeProvider> |
| 82 | + </PostHogProvider> |
| 83 | + ); |
| 84 | +} |
| 85 | + |
| 86 | +``` |
| 87 | + |
| 88 | +When you reload your app, you should see events autocaptured into PostHog. |
| 89 | + |
| 90 | +<ProductScreenshot |
| 91 | + imageLight="https://res.cloudinary.com/dmukukwp6/image/upload/Clean_Shot_2025_02_06_at_14_15_07_2x_c0c48ed6ad.png" |
| 92 | + imageDark="https://res.cloudinary.com/dmukukwp6/image/upload/Clean_Shot_2025_02_06_at_14_15_20_2x_59e92d7acb.png" |
| 93 | + alt="Events autocaptured in PostHog" |
| 94 | + classes="rounded" |
| 95 | +/> |
| 96 | + |
| 97 | +## 3. Creating a remote config in PostHog |
| 98 | + |
| 99 | +With PostHog set up, let's create a remote config flags to control a message in app: |
| 100 | + |
| 101 | +1. Go to the [feature flags tab](https://us.posthog.com/feature_flags) in PostHog and click **New feature flag** |
| 102 | +2. Enter `welcome-message` as the key |
| 103 | +3. Under **Served value**, select **Remote config (single payload)** |
| 104 | +4. Set the payload to a string `"Welcome to our awesome app!"` |
| 105 | +5. Click **Save** |
| 106 | + |
| 107 | +<ProductScreenshot |
| 108 | + imageLight="https://res.cloudinary.com/dmukukwp6/image/upload/Clean_Shot_2025_02_06_at_14_19_41_2x_717510a9a5.png" |
| 109 | + imageDark="https://res.cloudinary.com/dmukukwp6/image/upload/Clean_Shot_2025_02_06_at_14_19_27_2x_129ff2c847.png" |
| 110 | + alt="Creating a remote config in PostHog" |
| 111 | + classes="rounded" |
| 112 | +/> |
| 113 | + |
| 114 | +## 4. Creating and showing our remote config message |
| 115 | + |
| 116 | +Next, create a new file in your `components` directory named `RemoteConfigDemo.tsx`. In it, we will check the feature flag with `useFeatureFlagWithPayload` and display the message: |
| 117 | + |
| 118 | +```tsx |
| 119 | +import React from 'react'; |
| 120 | +import { StyleSheet, Text, View, useColorScheme } from 'react-native'; |
| 121 | +import { useFeatureFlagWithPayload } from 'posthog-react-native'; |
| 122 | +import { Colors } from '@/constants/Colors'; |
| 123 | + |
| 124 | +export default function RemoteConfigDemo() { |
| 125 | + const colorScheme = useColorScheme() ?? 'light'; |
| 126 | + const welcomeMessage = useFeatureFlagWithPayload('welcome-message') || 'Welcome!'; |
| 127 | + |
| 128 | + return ( |
| 129 | + <View style={styles.container}> |
| 130 | + <Text |
| 131 | + style={[ |
| 132 | + styles.text, |
| 133 | + { color: Colors[colorScheme].text } |
| 134 | + ]} |
| 135 | + > |
| 136 | + {welcomeMessage} |
| 137 | + </Text> |
| 138 | + </View> |
| 139 | + ); |
| 140 | +} |
| 141 | + |
| 142 | +const styles = StyleSheet.create({ |
| 143 | + container: { |
| 144 | + flex: 1, |
| 145 | + alignItems: 'center', |
| 146 | + justifyContent: 'center', |
| 147 | + }, |
| 148 | + logo: { |
| 149 | + width: 200, |
| 150 | + height: 200, |
| 151 | + marginBottom: 20, |
| 152 | + }, |
| 153 | + text: { |
| 154 | + fontSize: 20, |
| 155 | + }, |
| 156 | +}); |
| 157 | + |
| 158 | +``` |
| 159 | + |
| 160 | +Use this component in your home tab file (`app/(tabs)/index.tsx`) like this: |
| 161 | + |
| 162 | +```tsx |
| 163 | +import { StyleSheet } from 'react-native'; |
| 164 | +import { View } from '@/components/Themed'; |
| 165 | +import RemoteConfigDemo from '@/components/RemoteConfigDemo'; |
| 166 | + |
| 167 | +export default function TabOneScreen() { |
| 168 | + return ( |
| 169 | + <View style={styles.container}> |
| 170 | + <RemoteConfigDemo /> |
| 171 | + </View> |
| 172 | + ); |
| 173 | +} |
| 174 | + |
| 175 | +const styles = StyleSheet.create({ |
| 176 | + container: { |
| 177 | + flex: 1, |
| 178 | + alignItems: 'center', |
| 179 | + justifyContent: 'center', |
| 180 | + }, |
| 181 | +}); |
| 182 | +``` |
| 183 | + |
| 184 | +Finally, we can test our app by reloading or running `npx expo start` again and opening the Expo Go app. This will show the message from our remote config front and center. |
| 185 | + |
| 186 | +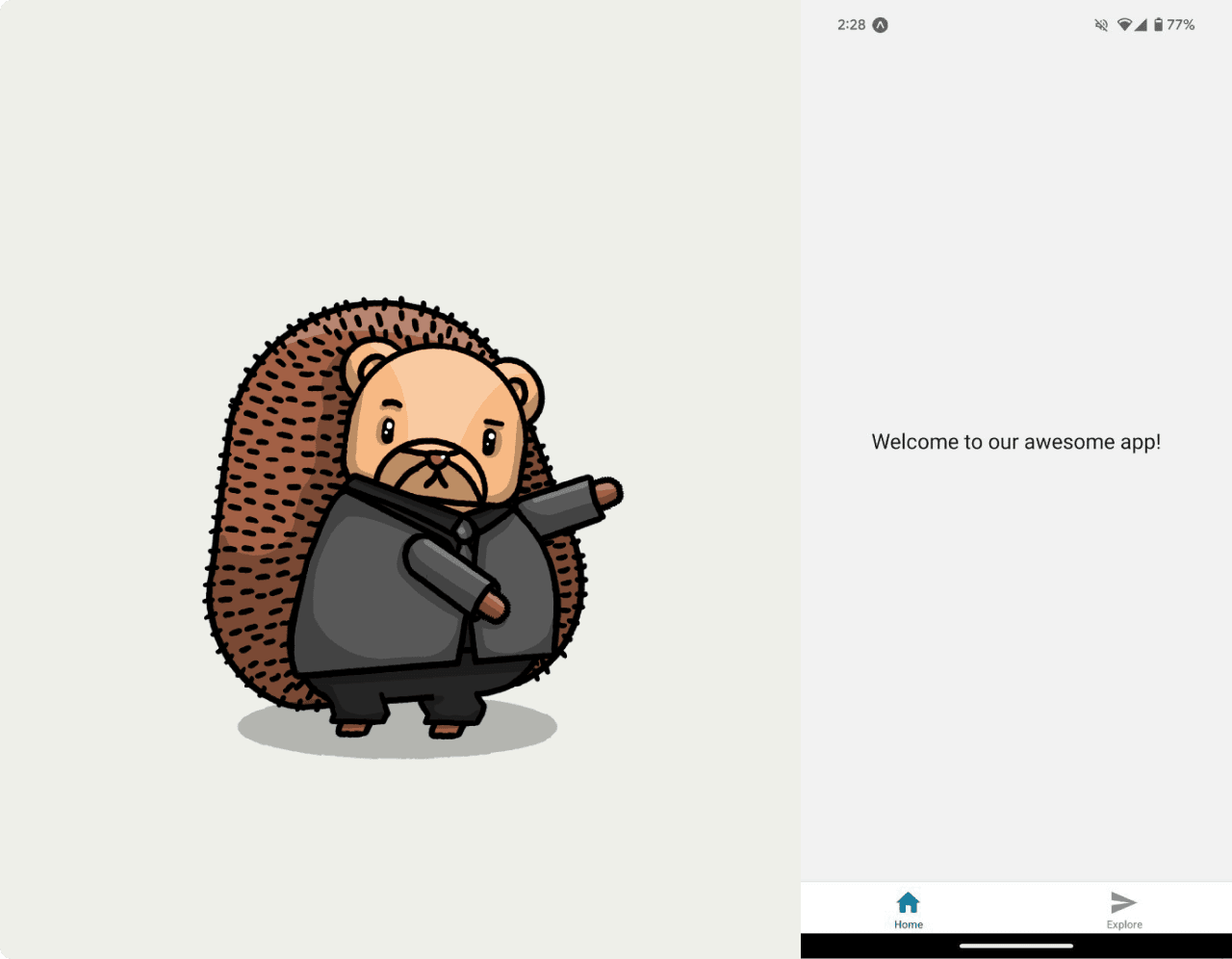 |
| 187 | + |
| 188 | +## Further reading |
| 189 | + |
| 190 | +- [Feature flags vs configuration: Which should you choose?](/product-engineers/feature-flags-vs-configuration) |
| 191 | +- [How to set up analytics in React Native](/tutorials/react-native-analytics) |
| 192 | +- [How to set up A/B tests in React Native](/react-native-ab-tests) |
0 commit comments