|
1 |
| -# DuckAndroidToast |
| 1 | +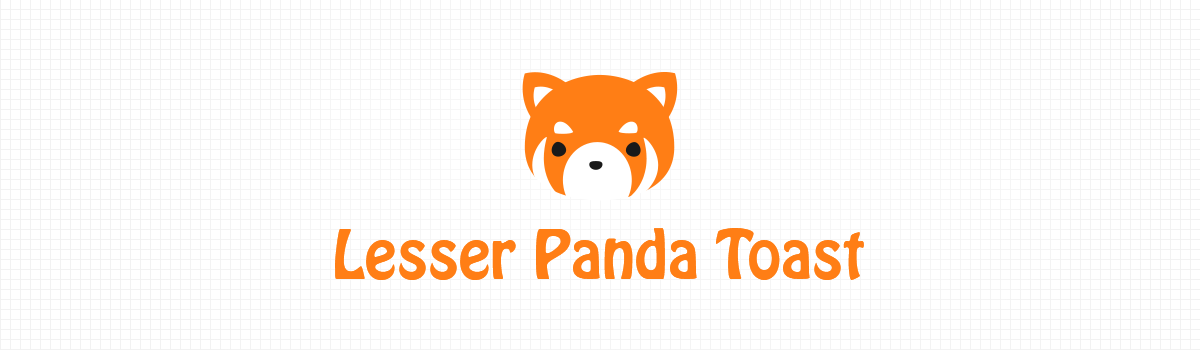 |
| 2 | + |
| 3 | +[](https://android-arsenal.com/api?level=14)  |
| 4 | +[](https://android-arsenal.com/api?level=14)  |
| 5 | +[](https://github.com/nasduck/LesserPandaToast/blob/master/LICENSE) |
| 6 | + |
| 7 | +LesserPandaToast provides the simplest solution to call different types of progresshuds or toasts and dismiss them in an indicated delay. Users could also customize their own styles. |
| 8 | + |
| 9 | +If you are also interested at Dialog. Please take a look at another library [GiantPandaDialog](https://github.com/nasduck/GiantPandaDialog). From these libraries' names, |
| 10 | +you will find they are used together. Please enjoy them :D |
| 11 | + |
| 12 | +## Content |
| 13 | + |
| 14 | +* [Setup](#setup) |
| 15 | +* [Usage](#usage) |
| 16 | + * [Show Toast](#show-toast) |
| 17 | + * [Dismiss Toast](#dismiss-toast) |
| 18 | + * [Custom Toast](#custom-toast) |
| 19 | +* [Contributer](#contributer) |
| 20 | +* [License](#license) |
| 21 | + |
| 22 | +## Setup |
| 23 | + |
| 24 | +### **</u>[中文文档](https://github.com/nasduck/LesserPandaToast/blob/master/README-CN.md)</u>** |
| 25 | + |
| 26 | +Adding jitpack repository in your project's `build.gradle` file: |
| 27 | + |
| 28 | +``` |
| 29 | +allprojects { |
| 30 | + repositories { |
| 31 | + ... |
| 32 | + maven { url 'https://www.jitpack.io' } |
| 33 | + } |
| 34 | +} |
| 35 | +``` |
| 36 | + |
| 37 | +Adding the following dependency to app `build.gradle` file: |
| 38 | + |
| 39 | + |
| 40 | +``` |
| 41 | +dependencies { |
| 42 | + implementation 'com.github.nasduck:LesserPandaToast:1.0.1' |
| 43 | +} |
| 44 | +``` |
| 45 | + |
| 46 | +## Usage |
| 47 | + |
| 48 | +5 types of Toast are provided by default: |
| 49 | + |
| 50 | +1. Text only |
| 51 | +2. Success |
| 52 | +3. Failure |
| 53 | +4. Warning |
| 54 | +5. Loading |
| 55 | + |
| 56 | +### Show Toast |
| 57 | + |
| 58 | +```java |
| 59 | +// Text only |
| 60 | +DuckToast.show(this, "Toast Default"); |
| 61 | + |
| 62 | +// Success |
| 63 | +DuckToast.showSuccess(this); // Image only |
| 64 | +DuckToast.showSuccess(this, "success"); // Image and text |
| 65 | + |
| 66 | +// Failure |
| 67 | +DuckToast.showFailure(this); // Image only |
| 68 | +DuckToast.showFailure(this, "failure"); // Image and text |
| 69 | + |
| 70 | +// Warning |
| 71 | +DuckToast.showWarning(this); // Image only |
| 72 | +DuckToast.showWarning(this, "warning"); // Image and text |
| 73 | + |
| 74 | +// Loading |
| 75 | +DuckToast.showLoading(this); // Image only |
| 76 | +DuckToast.showLoading(this, "loading"); // Image and text |
| 77 | +``` |
| 78 | + |
| 79 | +<img src="https://github.com/nasduck/LesserPandaToast/blob/develop/art/text%20toast.png?raw=true" height="300" > <img src="https://github.com/nasduck/LesserPandaToast/blob/develop/art/success%20toast.png?raw=true" height="300" > <img src="https://github.com/nasduck/LesserPandaToast/blob/develop/art/failure%20toast.png?raw=true" height="300" > <img src="https://github.com/nasduck/LesserPandaToast/blob/develop/art/warning%20toast.png?raw=true" height="300" > <img src="https://github.com/nasduck/LesserPandaToast/blob/develop/art/loading%20toast.gif?raw=true" height="300" > |
| 80 | + |
| 81 | +> After `showXXXX` is called, Toast will keep shown until user specifies how it will be dismissed. |
| 82 | +
|
| 83 | +### Dismiss Toast |
| 84 | + |
| 85 | +Two dismiss solution: |
| 86 | + |
| 87 | +1. Dismiss immediately |
| 88 | +2. Dismiss with delay |
| 89 | + |
| 90 | +```java |
| 91 | +DuckToast.dismiss(); // Dismiss immediately |
| 92 | +DuckToast.dismiss(long delay); // Dismiss with delay (ms) |
| 93 | +``` |
| 94 | + |
| 95 | +### Custom Toast |
| 96 | + |
| 97 | +Customize Toast: |
| 98 | + |
| 99 | +```java |
| 100 | +ToastBuilder.getInstance(this) |
| 101 | + .setImage(Integer image) // Image. If not set, other related image settings won't be enabled |
| 102 | + .setAnimation(Integer animation) // Image Animation |
| 103 | + .setBgColor(Integer bgColor) // Background color. #B2000000 by default |
| 104 | + .setCornerRadius(Integer cornerRadius) // Background corner radius. 6dp by default |
| 105 | + .setPaddingTop(Integer paddingTop) // 12dp by default |
| 106 | + .setPaddingBottom(Integer paddingBottom) // 12dp by default |
| 107 | + .setPaddingLeft(Integer paddingLeft) // 24dp by default |
| 108 | + .setPaddingRight(Integer paddingRight) // 24dp by default |
| 109 | + .setPaddingHorizontal(Integer paddingHorizontal) |
| 110 | + .setPaddingVertical(Integer paddingVertical) |
| 111 | + .setPadding(Integer padding) |
| 112 | + .setText(String text) // Text. If not set, other related text settings won't be enabled |
| 113 | + .setTextColor(Integer textColor) // white by default |
| 114 | + .setTextSize(Integer textSize) // 14sp by default |
| 115 | + .show(); |
| 116 | + |
| 117 | +ToastBuilder.dismiss(1500); // dismiss in 1.5s |
| 118 | +``` |
| 119 | + |
| 120 | +## Contributer |
| 121 | + |
| 122 | +* [Lihao Zhou](https://github.com/redrain39) |
| 123 | +* [Chuan DONG](https://github.com/DONGChuan) |
| 124 | +* [Si Cheng ]([email protected])(Art Designer) |
| 125 | + |
| 126 | +## LICENSE |
| 127 | +``` |
| 128 | + Copyright (2019) Chuan Dong, Lihao Zhou |
| 129 | +
|
| 130 | + Licensed under the Apache License, Version 2.0 (the "License"); |
| 131 | + you may not use this file except in compliance with the License. |
| 132 | + You may obtain a copy of the License at |
| 133 | +
|
| 134 | + http://www.apache.org/licenses/LICENSE-2.0 |
| 135 | +
|
| 136 | + Unless required by applicable law or agreed to in writing, software |
| 137 | + distributed under the License is distributed on an "AS IS" BASIS, |
| 138 | + WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
| 139 | + See the License for the specific language governing permissions and |
| 140 | + limitations under the License. |
| 141 | +``` |
0 commit comments