Commit 42cb260
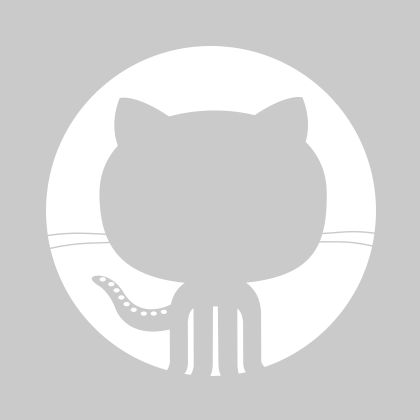
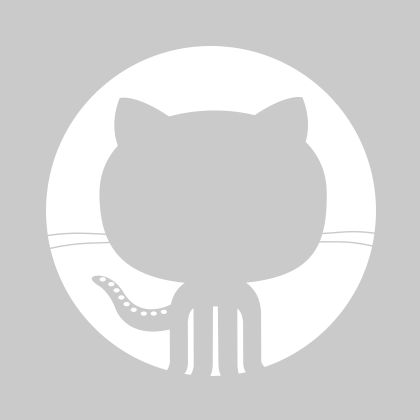
Benjamin Brienen
Benjamin Brienen
1 parent 080b942 commit 42cb260
File tree
21 files changed
+316
-172
lines changed- examples
- src
- green
- xtask
- src
- tests
21 files changed
+316
-172
lines changedLines changed: 9 additions & 8 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
9 |
| - | |
| 8 | + | |
| 9 | + | |
10 | 10 |
| |
11 | 11 |
| |
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
16 |
| - | |
17 |
| - | |
18 |
| - | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
19 | 20 |
| |
20 |
| - | |
21 |
| - | |
| 21 | + | |
| 22 | + | |
22 | 23 |
| |
23 |
| - | |
| 24 | + | |
24 | 25 |
| |
25 | 26 |
| |
26 | 27 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
9 |
| - | |
| 9 | + | |
10 | 10 |
| |
11 | 11 |
| |
12 | 12 |
| |
|
Lines changed: 1 addition & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
32 | 32 |
| |
33 | 33 |
| |
34 | 34 |
| |
| 35 | + | |
35 | 36 |
| |
36 | 37 |
| |
37 | 38 |
| |
|
Lines changed: 4 additions & 6 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
10 |
| - | |
| 10 | + | |
11 | 11 |
| |
12 | 12 |
| |
13 | 13 |
| |
| |||
194 | 194 |
| |
195 | 195 |
| |
196 | 196 |
| |
| 197 | + | |
197 | 198 |
| |
198 | 199 |
| |
| 200 | + | |
199 | 201 |
| |
200 | 202 |
| |
201 | 203 |
| |
| |||
255 | 257 |
| |
256 | 258 |
| |
257 | 259 |
| |
258 |
| - | |
259 |
| - | |
260 |
| - | |
261 |
| - | |
262 |
| - | |
| 260 | + | |
263 | 261 |
| |
264 | 262 |
| |
265 | 263 |
| |
|
Lines changed: 3 additions & 6 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 |
| - | |
| 4 | + | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| |||
446 | 446 |
| |
447 | 447 |
| |
448 | 448 |
| |
449 |
| - | |
450 |
| - | |
451 |
| - | |
452 |
| - | |
| 449 | + | |
453 | 450 |
| |
454 | 451 |
| |
455 | 452 |
| |
|
Lines changed: 10 additions & 12 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
8 | 8 |
| |
9 | 9 |
| |
10 | 10 |
| |
| |||
55 | 55 |
| |
56 | 56 |
| |
57 | 57 |
| |
58 |
| - | |
59 |
| - | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
60 | 62 |
| |
61 | 63 |
| |
62 | 64 |
| |
63 | 65 |
| |
64 | 66 |
| |
65 | 67 |
| |
| 68 | + | |
66 | 69 |
| |
67 | 70 |
| |
68 | 71 |
| |
| |||
74 | 77 |
| |
75 | 78 |
| |
76 | 79 |
| |
77 |
| - | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
78 | 83 |
| |
79 | 84 |
| |
80 | 85 |
| |
| |||
195 | 200 |
| |
196 | 201 |
| |
197 | 202 |
| |
198 |
| - | |
199 |
| - | |
200 |
| - | |
201 |
| - | |
202 | 203 |
| |
203 | 204 |
| |
204 | 205 |
| |
| |||
312 | 313 |
| |
313 | 314 |
| |
314 | 315 |
| |
315 |
| - | |
316 |
| - | |
317 |
| - | |
318 |
| - | |
| 316 | + | |
319 | 317 |
| |
320 | 318 |
| |
321 | 319 |
| |
|
Lines changed: 2 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
156 | 156 |
| |
157 | 157 |
| |
158 | 158 |
| |
159 |
| - | |
| 159 | + | |
160 | 160 |
| |
161 | 161 |
| |
162 | 162 |
| |
| |||
176 | 176 |
| |
177 | 177 |
| |
178 | 178 |
| |
179 |
| - | |
| 179 | + | |
180 | 180 |
| |
181 | 181 |
| |
182 | 182 |
| |
|
Lines changed: 2 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
12 |
| - | |
| 12 | + | |
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
| 21 | + | |
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
|
0 commit comments