|
| 1 | +--- |
| 2 | +icon: simple/arduino |
| 3 | +--- |
| 4 | + |
| 5 | +## Device Scan |
| 6 | +This sketch allows users to scan for devices connected to the primary I^2^C bus of the RAM5 Thing Plus. The example code can be found in the [GitHub repository](https://github.com/sparkfun/SparkFun_Thing_Plus_RA6M5/tree/main/docs/assets/arduino_examples/i2c_scanner/i2c_scanner.ino). However, users can also simply click on the button *(below)*, to download the code; or expand the box *(below)*, to copy the code. |
| 7 | + |
| 8 | + |
| 9 | +??? code "`i2c_scanner.ino`" |
| 10 | + ```cpp |
| 11 | + --8<-- "./assets/arduino_examples/i2c_scanner/i2c_scanner.ino" |
| 12 | + ``` |
| 13 | + |
| 14 | + |
| 15 | +<center> |
| 16 | +[:octicons-download-16:{ .heart } Download the Example Sketch](./assets/arduino_examples/i2c_scanner/i2c_scanner.ino){ .md-button .md-button--primary } |
| 17 | +</center> |
| 18 | + |
| 19 | + |
| 20 | +## Peripheral Devices |
| 21 | +The RA6M5 Thing Plus features a Qwiic connector to seamlessly integrate with devices from [SparkFun's Qwiic Ecosystem](https://www.sparkfun.com/qwiic). While users are free to utilize any I^2^C device, we recommend the [Qwiic devices](https://www.sparkfun.com/categories/399) from our catalog. |
| 22 | + |
| 23 | +??? note "Optional Hardware" |
| 24 | + <div class="grid cards" markdown> |
| 25 | + |
| 26 | + - <a href="https://www.sparkfun.com/products/15081"> |
| 27 | + <figure markdown> |
| 28 | + 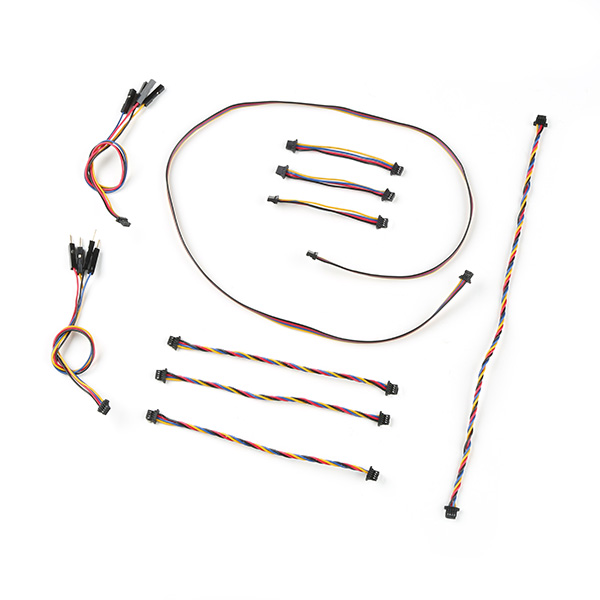 |
| 29 | + </figure> |
| 30 | + |
| 31 | + --- |
| 32 | + |
| 33 | + **SparkFun Qwiic Cable Kit**<br> |
| 34 | + KIT-15081</a> |
| 35 | + |
| 36 | + |
| 37 | + - <a href="https://www.sparkfun.com/products/15109"> |
| 38 | + <figure markdown> |
| 39 | + 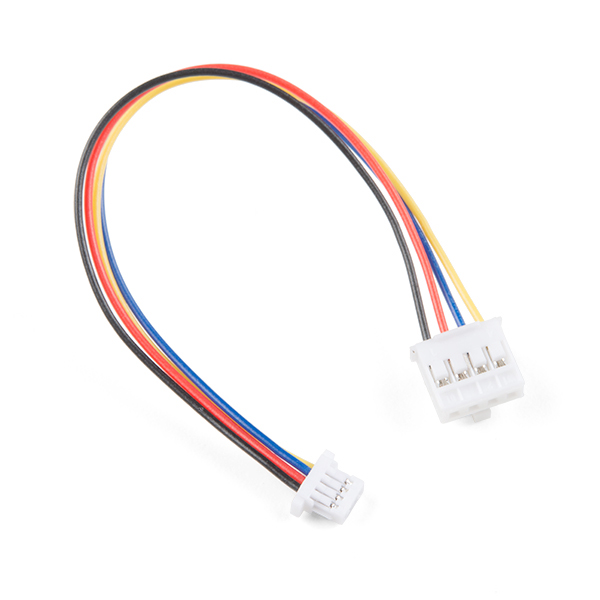 |
| 40 | + </figure> |
| 41 | + |
| 42 | + --- |
| 43 | + |
| 44 | + **Qwiic Cable - Grove Adapter (100mm)**<br> |
| 45 | + PRT-15109</a> |
| 46 | + |
| 47 | + |
| 48 | + - <a href="https://www.sparkfun.com/products/23453"> |
| 49 | + <figure markdown> |
| 50 | + 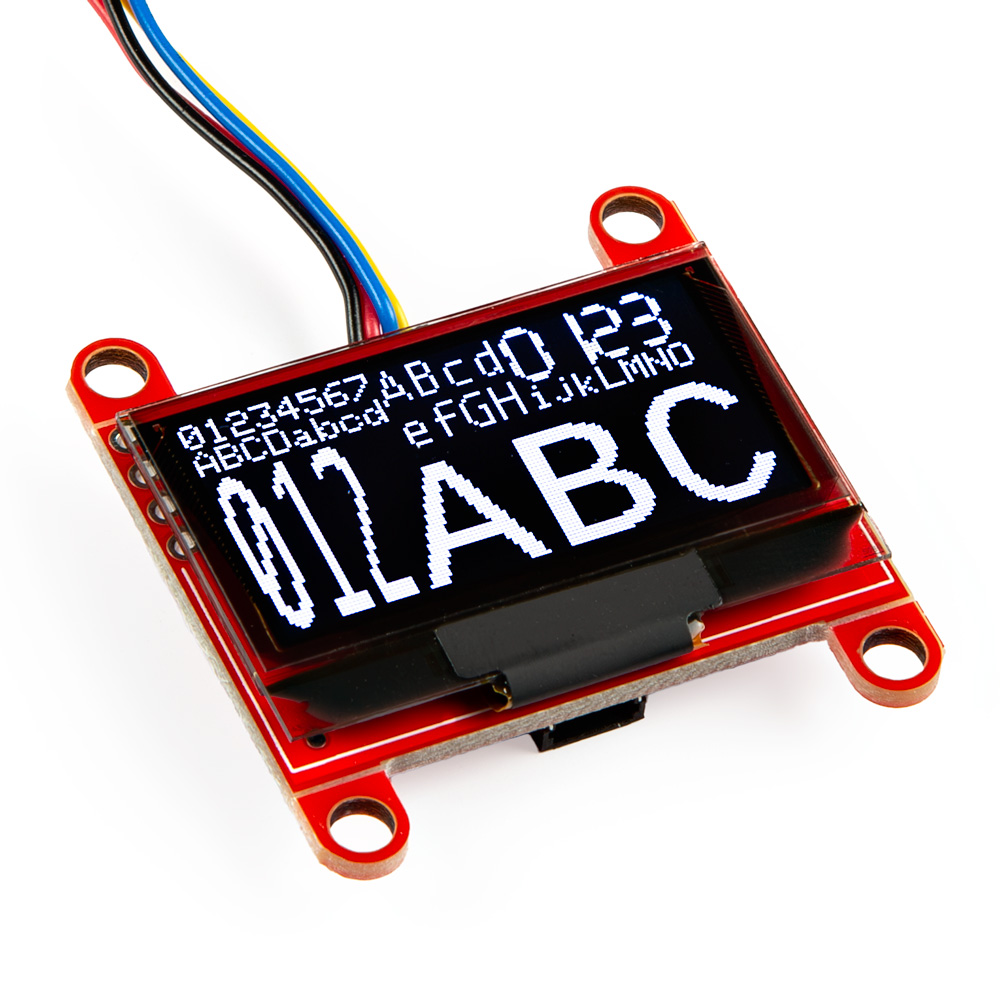 |
| 51 | + </figure> |
| 52 | + |
| 53 | + --- |
| 54 | + |
| 55 | + **SparkFun Qwiic OLED - (1.3in., 128x64)**<br> |
| 56 | + LCD-23453</a> |
| 57 | + |
| 58 | + |
| 59 | + - <a href="https://www.sparkfun.com/products/14414"> |
| 60 | + <figure markdown> |
| 61 | + 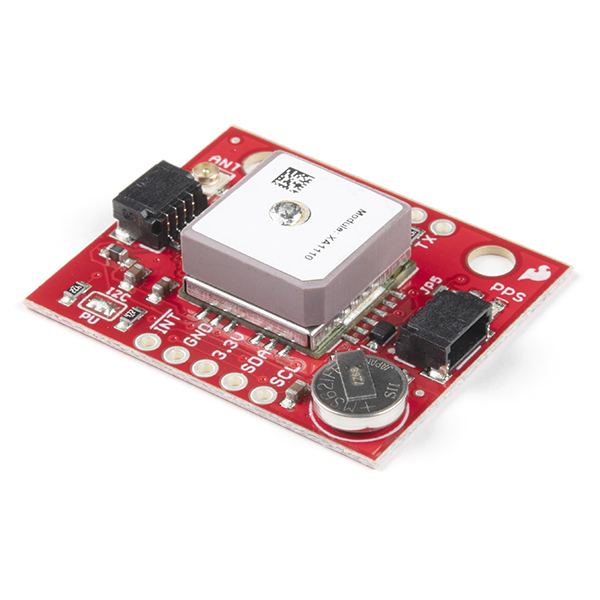 |
| 62 | + </figure> |
| 63 | + |
| 64 | + --- |
| 65 | + |
| 66 | + **SparkFun GPS Breakout - XA1110 (Qwiic)**<br> |
| 67 | + GPS-14414</a> |
| 68 | + |
| 69 | + |
| 70 | + - <a href="https://www.sparkfun.com/products/15168"> |
| 71 | + <figure markdown> |
| 72 | + 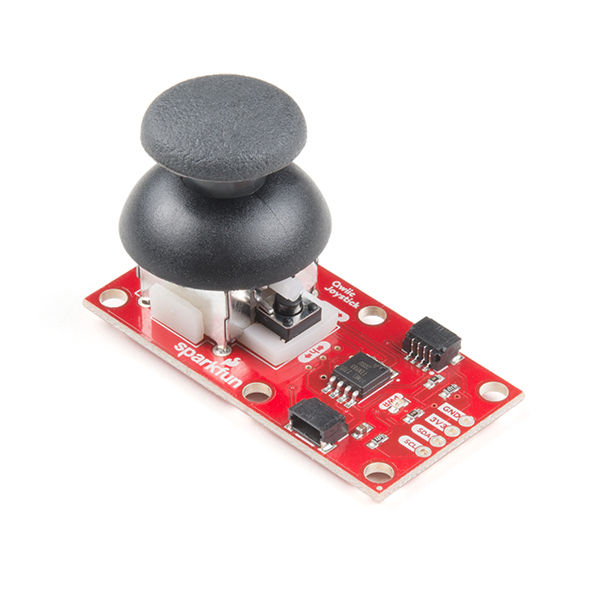 |
| 73 | + </figure> |
| 74 | + |
| 75 | + --- |
| 76 | + |
| 77 | + **SparkFun Qwiic Joystick**<br> |
| 78 | + COM-15168</a> |
| 79 | + |
| 80 | + |
| 81 | + - <a href="https://www.sparkfun.com/products/16784"> |
| 82 | + <figure markdown> |
| 83 | + 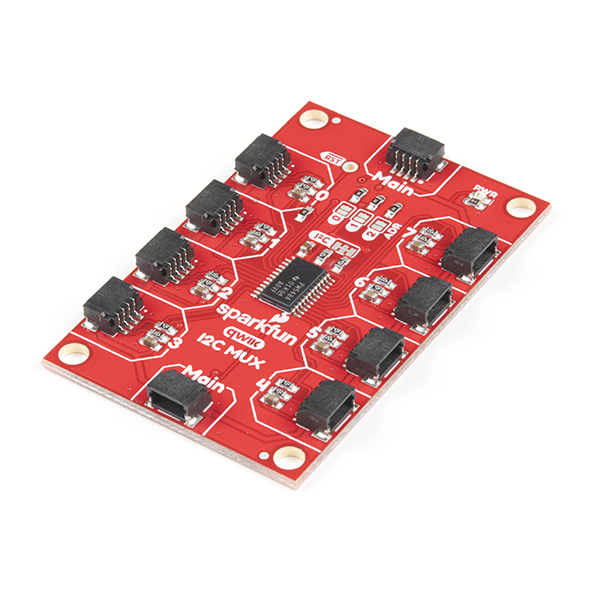 |
| 84 | + </figure> |
| 85 | + |
| 86 | + --- |
| 87 | + |
| 88 | + **SparkFun Qwiic Mux Breakout - 8 Channel (TCA9548A)**<br> |
| 89 | + BOB-16784</a> |
| 90 | + |
| 91 | + |
| 92 | + - <a href="https://www.sparkfun.com/products/19096"> |
| 93 | + <figure markdown> |
| 94 | + 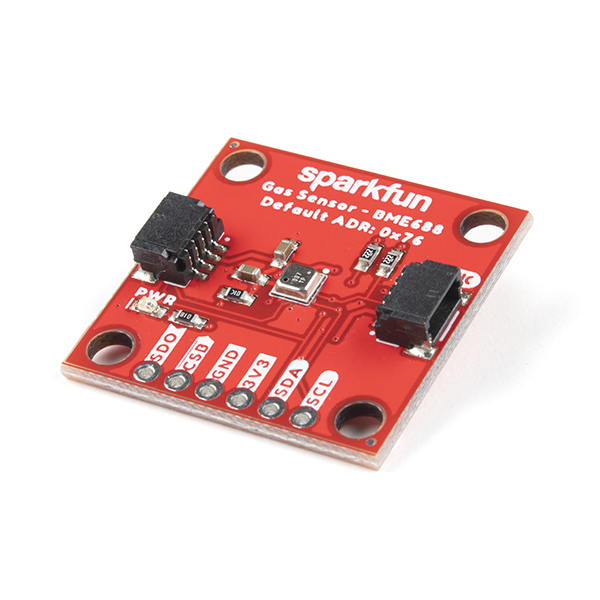 |
| 95 | + </figure> |
| 96 | + |
| 97 | + --- |
| 98 | + |
| 99 | + **SparkFun Environmental Sensor - BME688 (Qwiic)**<br> |
| 100 | + SEN-19096</a> |
| 101 | + |
| 102 | + |
| 103 | + - <a href="https://www.sparkfun.com/products/19013"> |
| 104 | + <figure markdown> |
| 105 | + 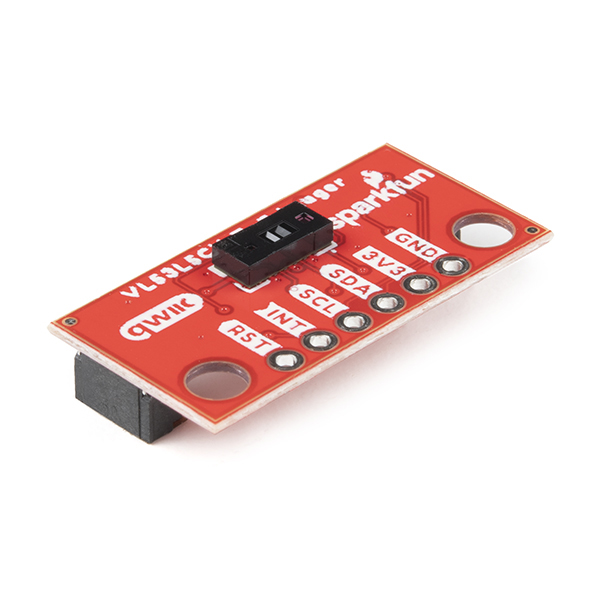 |
| 106 | + </figure> |
| 107 | + |
| 108 | + --- |
| 109 | + |
| 110 | + **SparkFun Qwiic Mini ToF Imager - VL53L5CX**<br> |
| 111 | + SEN-19013</a> |
| 112 | + |
| 113 | + </div> |
| 114 | + |
| 115 | + |
| 116 | +### MAX17048 Fuel Gauge |
| 117 | +The MAX17048 fuel gauge measures the approximate charge/discharge rate, state of charge, and voltage of a connected LiPo battery. We recommend the [SparkFun MAX1704x Arduino library](https://github.com/sparkfun/SparkFun_MAX1704x_Fuel_Gauge_Arduino_Library) be utilized in the Arduino IDE, to connect to the MAX17048 on the RA6M5 Thing Plus. Once the [library is installed in the Arduino IDE](../software_overview-arduino/#max17048-fuel-gauge), users will find several example sketches listed in the **File** > **Examples** > **SparkFun MAX1704x Fuel Gauge Arduino Library** > drop-down menu. We recommend the following examples for users: |
| 118 | + |
| 119 | +- `Example1_Simple.ino` |
| 120 | +- `Example4_MAX17048_KitchenSink.ino` |
| 121 | + |
| 122 | +??? note "Optional Hardware" |
| 123 | + <div class="grid cards" markdown> |
| 124 | + |
| 125 | + - <a href="https://www.sparkfun.com/products/13855"> |
| 126 | + <figure markdown> |
| 127 | + 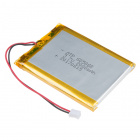 |
| 128 | + </figure> |
| 129 | + |
| 130 | + --- |
| 131 | + |
| 132 | + **Lithium Ion Battery - 2Ah**<br> |
| 133 | + PRT-13855</a> |
| 134 | + |
| 135 | + |
| 136 | + - <a href="https://www.sparkfun.com/products/13851"> |
| 137 | + <figure markdown> |
| 138 | + 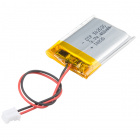 |
| 139 | + </figure> |
| 140 | + |
| 141 | + --- |
| 142 | + |
| 143 | + **Lithium Ion Battery - 400mAh**<br> |
| 144 | + PRT-13851</a> |
| 145 | + |
| 146 | + |
| 147 | + - <a href="https://www.sparkfun.com/products/13813"> |
| 148 | + <figure markdown> |
| 149 | + 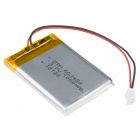 |
| 150 | + </figure> |
| 151 | + |
| 152 | + --- |
| 153 | + |
| 154 | + **Lithium Ion Battery - 1Ah**<br> |
| 155 | + PRT-13813</a> |
| 156 | + |
| 157 | + |
| 158 | + - <a href="https://www.sparkfun.com/products/13853"> |
| 159 | + <figure markdown> |
| 160 | + 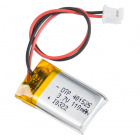 |
| 161 | + </figure> |
| 162 | + |
| 163 | + --- |
| 164 | + |
| 165 | + **Lithium Ion Battery - 110mAh**<br> |
| 166 | + PRT-13853</a> |
| 167 | + |
| 168 | + </div> |
| 169 | + |
| 170 | + |
| 171 | +=== "`Example1_Simple.ino`" |
| 172 | + Users can find this sketch in the **File** > **Examples** > **SparkFun MAX1704x Fuel Gauge Arduino Library** > **Example1_Simple** drop-down menu. |
| 173 | + |
| 174 | + ??? code "`Example1_Simple.ino`" |
| 175 | + ```cpp |
| 176 | + --8<-- "https://raw.githubusercontent.com/sparkfun/SparkFun_MAX1704x_Fuel_Gauge_Arduino_Library/main/examples/Example1_Simple/Example1_Simple.ino" |
| 177 | + ``` |
| 178 | + |
| 179 | + |
| 180 | +=== "`Example4_MAX17048_KitchenSink.ino`" |
| 181 | + Users can find this sketch in the **File** > **Examples** > **SparkFun MAX1704x Fuel Gauge Arduino Library** > **Example4_MAX17048_KitchenSink** drop-down menu. |
| 182 | + |
| 183 | + ??? code "`Example4_MAX17048_KitchenSink.ino`" |
| 184 | + ```cpp |
| 185 | + --8<-- "https://raw.githubusercontent.com/sparkfun/SparkFun_MAX1704x_Fuel_Gauge_Arduino_Library/main/examples/Example4_MAX17048_KitchenSink/Example4_MAX17048_KitchenSink.ino" |
| 186 | + ``` |
0 commit comments