|
| 1 | +# 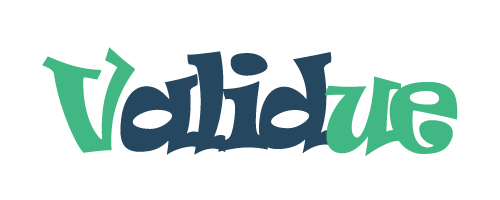 |
| 2 | + |
| 3 | +This library is based on the "[class-validator](https://github.com/typestack/class-validator)" library. It will help you easily validate your fields, forms and etc.. All you need to use is @PropertyValidator , @ErrorValidator, @ActionValidator decorators, and all decorators from “class validator” such as @Max, @IsEmail and etc.. |
| 4 | + |
| 5 | +# Instalation |
| 6 | + |
| 7 | + npm i validue |
| 8 | + |
| 9 | + |
| 10 | +# Demo |
| 11 | +Go to the link bellow for watch demo |
| 12 | + |
| 13 | +> [Link demo on the codesandbox](https://codesandbox.io/s/headless-wave-1ri0c?file=/src/App.vue) |
| 14 | +
|
| 15 | +# Example |
| 16 | + |
| 17 | +Validation errors check automatically because Validue create a Vue Watcher for watching field editing. This |
| 18 | +example close to the real task, all you need to append for work it's a business logic in the SignUp method |
| 19 | + |
| 20 | +```vue |
| 21 | +<template> |
| 22 | + <form> |
| 23 | + <div class="text-field"> |
| 24 | + <label class="text-field__label" for="username"> Your username </label> |
| 25 | + <input class="text-field__input" id="username" placeholder="Enter username"> |
| 26 | + <p class="text-field__error" v-if="usernameErrors.isNotEmpty || usernameErrors.length"> |
| 27 | + {{usernameErrors.isNotEmpty || usernameErrors.length}} |
| 28 | + </p> |
| 29 | + </div> |
| 30 | + <div class="text-field"> |
| 31 | + <label class="text-field__label" for="email"> Your email </label> |
| 32 | + <input class="text-field__input" id="email" placeholder="Enter email"> |
| 33 | + <p class="text-field__error" v-if="emailErrors.isNotEmpty || emailErrors.isEmail"> |
| 34 | + {{emailErrors.isNotEmpty || emailErrors.isEmail}} |
| 35 | + </p> |
| 36 | + </div> |
| 37 | + <button @click="signUp"> Sign up </button> |
| 38 | + </form> |
| 39 | +</template> |
| 40 | +<script lang="ts"> |
| 41 | +import {Component, Vue} from "vue-property-decorator"; |
| 42 | +import {ActionValidator, PropertyValidator, ErrorValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 43 | +
|
| 44 | +@Component({}) |
| 45 | +export default class App extends Vue { |
| 46 | +
|
| 47 | + @IsNotEmpty({message: "Required field"}) |
| 48 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 49 | + username = ""; |
| 50 | +
|
| 51 | + @IsNotEmpty({message: "Required field"}) |
| 52 | + @IsEmail({}, {message: "Wrong email address"}) |
| 53 | + email = ""; |
| 54 | +
|
| 55 | + @PropertyValidator("username") |
| 56 | + usernameErrors = {}; |
| 57 | +
|
| 58 | + @PropertyValidator("email") |
| 59 | + emailErrors = {}; |
| 60 | + |
| 61 | + @ActionValidator() |
| 62 | + signUp(@ErrorValidator errors: []){ |
| 63 | + if(errors.length){ |
| 64 | + console.log('U have some errors'); |
| 65 | + return; |
| 66 | + } |
| 67 | + //... your business logic |
| 68 | + } |
| 69 | +} |
| 70 | +</script> |
| 71 | +``` |
| 72 | + |
| 73 | +## Usage |
| 74 | +### @PropertyValidator has 3 override syntax: |
| 75 | +#### PropertyValidator(path: string, validationFunctions?: Function[], options?: WatchOptions) |
| 76 | +#### PropertyValidator(path: string, validationFunctions?:Function[]) |
| 77 | +#### PropertyValidator(path: string, options?: WatchOptions) |
| 78 | + |
| 79 | + |
| 80 | +You need to add this decorator before the field, errors in which are written after validation. First argument is a field that is watched. It works like @Watch decorator in Vue. Second argument receives an array of validation functions. This argument is not required because you can use 2 methods to declare validation of your fields. |
| 81 | + |
| 82 | +> All decorators of "class-validator" in [here](https://github.com/typestack/class-validator#validation-decorators) |
| 83 | +
|
| 84 | +Example: |
| 85 | +1 Way: |
| 86 | +```typescript |
| 87 | + import {Component, Vue} from "vue-property-decorator"; |
| 88 | + import {ActionValidator, PropertyValidator,IsNotEmpty, IsEmail, Length} from "validue"; |
| 89 | + |
| 90 | + @Component({}) |
| 91 | + export default class App extends Vue { |
| 92 | + |
| 93 | + @IsNotEmpty({message: "Required field"}) |
| 94 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 95 | + firstName = ""; |
| 96 | + |
| 97 | + @IsNotEmpty({message: "Required field"}) |
| 98 | + @IsEmail({}, {message: "Wrong email address"}) |
| 99 | + email = ""; |
| 100 | + |
| 101 | + @PropertyValidator("firstName") |
| 102 | + firstNameErrors = {}; |
| 103 | + |
| 104 | + @PropertyValidator("email") |
| 105 | + emailErrors = {}; |
| 106 | + } |
| 107 | +``` |
| 108 | + |
| 109 | +2 Way: |
| 110 | + |
| 111 | + ```typescript |
| 112 | + |
| 113 | + import {Component, Vue} from "vue-property-decorator"; |
| 114 | + import {ActionValidator, PropertyValidator,IsNotEmpty, IsEmail, Length} from "validue"; |
| 115 | + |
| 116 | + @Component({}) |
| 117 | + export default class App extends Vue { |
| 118 | + |
| 119 | + firstName = ""; |
| 120 | + email = ""; |
| 121 | + |
| 122 | + @PropertyValidator("firstName", [ |
| 123 | + IsNotEmpty({message: "Required field"}), |
| 124 | + Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 125 | + ]) |
| 126 | + firstNameErrors = {}; |
| 127 | + |
| 128 | + @PropertyValidator("email", [ |
| 129 | + IsNotEmpty({message: "Required field"}), |
| 130 | + IsEmail({}, {message: "Wrong email address"}) |
| 131 | + ]) |
| 132 | + emailErrors = {}; |
| 133 | + } |
| 134 | +``` |
| 135 | + |
| 136 | +### @ActionValidator(group?: string[]) |
| 137 | +This decorator is added before the method. Thus, before the method is called, all fields and field groups are validated, and returned errors are written in fields with @ProperyValidator added before. |
| 138 | +Example without group: |
| 139 | +```typescript |
| 140 | + import {Component, Vue} from "vue-property-decorator"; |
| 141 | + import {ActionValidator, PropertyValidator,IsNotEmpty, IsEmail, Length} from "validue"; |
| 142 | + |
| 143 | + @Component({}) |
| 144 | + export default class App extends Vue { |
| 145 | + |
| 146 | + @IsNotEmpty({message: "Required field"}) |
| 147 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 148 | + firstName = ""; |
| 149 | + |
| 150 | + @IsNotEmpty({message: "Required field"}) |
| 151 | + @IsEmail({}, {message: "Wrong email address"}) |
| 152 | + email = ""; |
| 153 | + |
| 154 | + @PropertyValidator("firstName") |
| 155 | + firstNameErrors = {}; |
| 156 | + |
| 157 | + @PropertyValidator("email") |
| 158 | + emailErrors = {}; |
| 159 | + |
| 160 | + @ActionValidator() |
| 161 | + send(){ |
| 162 | + console.log(this.firstNameErrors, this.emailErrors); |
| 163 | + } |
| 164 | + } |
| 165 | +``` |
| 166 | + |
| 167 | +Example with group: |
| 168 | +```typescript |
| 169 | + import {Component, Vue} from "vue-property-decorator"; |
| 170 | + import {ActionValidator, PropertyValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 171 | + |
| 172 | + @Component({}) |
| 173 | + export default class App extends Vue { |
| 174 | + |
| 175 | + @IsNotEmpty({message: "Required field", |
| 176 | + groups: ['registration']}) |
| 177 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char", |
| 178 | + groups: ['registration']}) |
| 179 | + firstName = ""; |
| 180 | + |
| 181 | + @IsNotEmpty({message: "Required field", |
| 182 | + groups: ['registration']}) |
| 183 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char", |
| 184 | + groups: ['registration']}) |
| 185 | + lastName = ""; |
| 186 | + |
| 187 | + @PropertyValidator("firstName") |
| 188 | + firstNameErrors = {}; |
| 189 | + |
| 190 | + @PropertyValidator("lastName") |
| 191 | + lastNameErrors = {}; |
| 192 | + |
| 193 | + @IsNotEmpty({message: "Required field", |
| 194 | + groups: ['auth']}) |
| 195 | + @IsEmail({}, {message: "Wrong email address", groups: ['auth']}) |
| 196 | + email = ""; |
| 197 | + |
| 198 | + @IsNotEmpty({message: "Required field", |
| 199 | + groups: ['auth']}) |
| 200 | + @IsEmail({}, {message: "Wrong email address", groups: ['auth']}) |
| 201 | + password = ""; |
| 202 | + |
| 203 | + @PropertyValidator("email") |
| 204 | + emailErrors = {}; |
| 205 | + |
| 206 | + @PropertyValidator("password") |
| 207 | + passwordErrors = {}; |
| 208 | + |
| 209 | + @ActionValidator(['registration']) |
| 210 | + register(){ |
| 211 | + //Will validate fields which have group 'registration' |
| 212 | + } |
| 213 | + |
| 214 | + @ActionValidator(['auth']) |
| 215 | + auth(){ |
| 216 | + //Will validate fields which have group 'auth' |
| 217 | + } |
| 218 | + } |
| 219 | +``` |
| 220 | + |
| 221 | +> All decorators of "class-validator" in [here](https://github.com/typestack/class-validator#validation-decorators) |
| 222 | +
|
| 223 | +Example: |
| 224 | +1 Way: |
| 225 | +```typescript |
| 226 | + import {Component, Vue} from "vue-property-decorator"; |
| 227 | + import {ActionValidator, PropertyValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 228 | + |
| 229 | + @Component({}) |
| 230 | + export default class App extends Vue { |
| 231 | + |
| 232 | + @IsNotEmpty({message: "Required field"}) |
| 233 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 234 | + firstName = ""; |
| 235 | + |
| 236 | + @IsNotEmpty({message: "Required field"}) |
| 237 | + @IsEmail({}, {message: "Wrong email address"}) |
| 238 | + email = ""; |
| 239 | + |
| 240 | + @PropertyValidator("firstName") |
| 241 | + firstNameErrors = {}; |
| 242 | + |
| 243 | + @PropertyValidator("email") |
| 244 | + emailErrors = {}; |
| 245 | + } |
| 246 | +``` |
| 247 | + |
| 248 | +2 Way: |
| 249 | + |
| 250 | + ```typescript |
| 251 | + |
| 252 | + import {Component, Vue} from "vue-property-decorator"; |
| 253 | + import {ActionValidator, PropertyValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 254 | + |
| 255 | + @Component({}) |
| 256 | + export default class App extends Vue { |
| 257 | + |
| 258 | + firstName = ""; |
| 259 | + email = ""; |
| 260 | + |
| 261 | + @PropertyValidator("firstName", [ |
| 262 | + IsNotEmpty({message: "Required field"}), |
| 263 | + Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 264 | + ]) |
| 265 | + firstNameErrors = {}; |
| 266 | + |
| 267 | + @PropertyValidator("email", [ |
| 268 | + IsNotEmpty({message: "Required field"}), |
| 269 | + IsEmail({}, {message: "Wrong email address"}) |
| 270 | + ]) |
| 271 | + emailErrors = {}; |
| 272 | + } |
| 273 | +``` |
| 274 | + |
| 275 | +### @ActionValidator(group?: string[]) |
| 276 | +This decorator is added before the method. Thus, before the method is called, all fields and field groups are validated, and returned errors are written in fields with @ProperyValidator added before. |
| 277 | +Example without group: |
| 278 | +```typescript |
| 279 | + import {Component, Vue} from "vue-property-decorator"; |
| 280 | + import {ActionValidator, PropertyValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 281 | + |
| 282 | + @Component({}) |
| 283 | + export default class App extends Vue { |
| 284 | + |
| 285 | + @IsNotEmpty({message: "Required field"}) |
| 286 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 287 | + firstName = ""; |
| 288 | + |
| 289 | + @IsNotEmpty({message: "Required field"}) |
| 290 | + @IsEmail({}, {message: "Wrong email address"}) |
| 291 | + email = ""; |
| 292 | + |
| 293 | + @PropertyValidator("firstName") |
| 294 | + firstNameErrors = {}; |
| 295 | + |
| 296 | + @PropertyValidator("email") |
| 297 | + emailErrors = {}; |
| 298 | + |
| 299 | + @ActionValidator() |
| 300 | + send(){ |
| 301 | + console.log(this.firstNameErrors, this.emailErrors); |
| 302 | + } |
| 303 | + } |
| 304 | +``` |
| 305 | + |
| 306 | +Example with group: |
| 307 | +```typescript |
| 308 | + import {Component, Vue} from "vue-property-decorator"; |
| 309 | +import {ActionValidator, PropertyValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 310 | + |
| 311 | +@Component({}) |
| 312 | +export default class App extends Vue { |
| 313 | + |
| 314 | + @IsNotEmpty({message: "Required field", |
| 315 | + groups: ['registration']}) |
| 316 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char", |
| 317 | + groups: ['registration']}) |
| 318 | + firstName = ""; |
| 319 | + |
| 320 | + @IsNotEmpty({message: "Required field", |
| 321 | + groups: ['registration']}) |
| 322 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char", |
| 323 | + groups: ['registration']}) |
| 324 | + lastName = ""; |
| 325 | + |
| 326 | + @PropertyValidator("firstName") |
| 327 | + firstNameErrors = {}; |
| 328 | + |
| 329 | + @PropertyValidator("lastName") |
| 330 | + lastNameErrors = {}; |
| 331 | + |
| 332 | + @IsNotEmpty({message: "Required field", |
| 333 | + groups: ['auth']}) |
| 334 | + @IsEmail({}, {message: "Wrong email address", groups: ['auth']}) |
| 335 | + email = ""; |
| 336 | + |
| 337 | + @IsNotEmpty({message: "Required field", |
| 338 | + groups: ['auth']}) |
| 339 | + @IsEmail({}, {message: "Wrong email address", groups: ['auth']}) |
| 340 | + password = ""; |
| 341 | + |
| 342 | + @PropertyValidator("email") |
| 343 | + emailErrors = {}; |
| 344 | + |
| 345 | + @PropertyValidator("password") |
| 346 | + passwordErrors = {}; |
| 347 | + |
| 348 | + @ActionValidator(['registration']) |
| 349 | + register(){ |
| 350 | + //Will validate fields which have group 'registration' |
| 351 | + } |
| 352 | + |
| 353 | + @ActionValidator(['auth']) |
| 354 | + auth(){ |
| 355 | + //Will validate fields which have group 'auth' |
| 356 | + } |
| 357 | +} |
| 358 | +``` |
| 359 | + |
| 360 | +### @ErrorValidator paramName |
| 361 | +This decorator is added in method's param and after call the method in this variable will write all need errors ('need' cuz u can use Groups, see upper) |
| 362 | + |
| 363 | +```typescript |
| 364 | +import {Component, Vue} from "vue-property-decorator"; |
| 365 | +import {ActionValidator, PropertyValidator, IsNotEmpty, IsEmail, Length} from "validue"; |
| 366 | +import {ErrorValidator} from './validue-decorators'; |
| 367 | + |
| 368 | +@Component({}) |
| 369 | +export default class App extends Vue { |
| 370 | + |
| 371 | + @IsNotEmpty({message: "Required field"}) |
| 372 | + @Length(1, 10, {message: "Field more then 10 chars or less then 1 char"}) |
| 373 | + firstName = ""; |
| 374 | + |
| 375 | + @IsNotEmpty({message: "Required field"}) |
| 376 | + @IsEmail({}, {message: "Wrong email address"}) |
| 377 | + email = ""; |
| 378 | + |
| 379 | + @PropertyValidator("firstName") |
| 380 | + firstNameErrors = {}; |
| 381 | + |
| 382 | + @PropertyValidator("email") |
| 383 | + emailErrors = {}; |
| 384 | + |
| 385 | + @ActionValidator() |
| 386 | + send(@ErrorValidator errors) { |
| 387 | + console.log(errors) // errors = [ {firstNameErrors: {...errors-here...}}, {emailErrors: {...errors-here...}}] |
| 388 | + } |
| 389 | +} |
| 390 | +``` |
0 commit comments