Commit 905324f
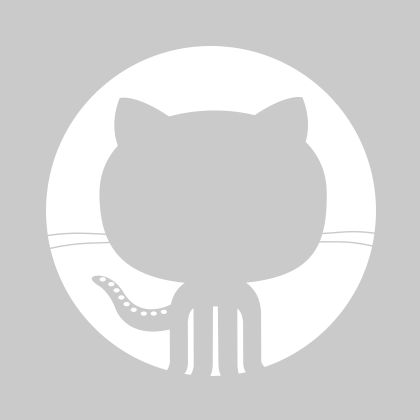
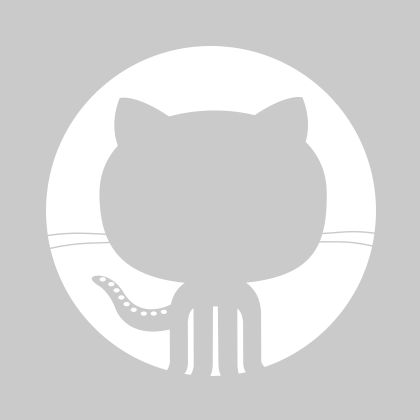
Max Batischev
Max Batischev
1 parent bf478d9 commit 905324f
File tree
4 files changed
+75
-0
lines changed- core/src
- main/java/org/springframework/security/authorization/method
- test/java/org/springframework/security/authorization/method
4 files changed
+75
-0
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
50 | 55 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
28 | 28 |
| |
29 | 29 |
| |
30 | 30 |
| |
| 31 | + | |
31 | 32 |
| |
32 | 33 |
| |
33 | 34 |
| |
| |||
64 | 65 |
| |
65 | 66 |
| |
66 | 67 |
| |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
67 | 72 |
| |
68 | 73 |
| |
69 | 74 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
21 | 21 |
| |
22 | 22 |
| |
23 | 23 |
| |
| 24 | + | |
24 | 25 |
| |
25 | 26 |
| |
26 | 27 |
| |
| |||
31 | 32 |
| |
32 | 33 |
| |
33 | 34 |
| |
| 35 | + | |
34 | 36 |
| |
35 | 37 |
| |
36 | 38 |
| |
| |||
156 | 158 |
| |
157 | 159 |
| |
158 | 160 |
| |
| 161 | + | |
| 162 | + | |
| 163 | + | |
| 164 | + | |
| 165 | + | |
| 166 | + | |
| 167 | + | |
| 168 | + | |
| 169 | + | |
| 170 | + | |
159 | 171 |
| |
160 | 172 |
| |
161 | 173 |
| |
| |||
176 | 188 |
| |
177 | 189 |
| |
178 | 190 |
| |
| 191 | + | |
179 | 192 |
| |
180 | 193 |
| |
181 | 194 |
| |
|
0 commit comments